This blog continues our series of C# coding brainteasers, which teach you how to read code without compiling it. This blog will provide 5 puzzles on Arrays. The puzzles will help you to learn practical programming skills.
An array is a container object of values of a single type. The length of array is established when array is created and it’s length is fixed. In order to know more how to use arrays please go to C# tutorial.
Try to solve these 5 arrays with your C# or other coding knowledge. Predict the output without executing the code. What language feature or standard algorithm do they illustrate? Is there more effective way that produces the same output? If you need some help, check our C# course. Write your answer in the comments below. Or add your own puzzle.
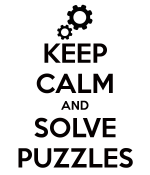
1).
int[] a = { 5, 3, 2 };
int m = a[0];
if (m > a[1])
{
m = a[1];
}
if (m > a[2])
{
m = a[2];
}
Console.WriteLine(m);
Console.ReadLine();
2).
int[] b = { 9, 8, 7, 10, 4, 6, 5, 1, 3, 2 };
int temp;
for (int i = 0; i < 9; i++)
{
for (int j = 0; j < 9-i; j++)
{
if (b[j] < b[j + 1])
{
temp = b[j];
b[j] = b[j + 1];
b[j + 1] = temp;
}
}
}
for (int i = 0; i < 10; i++)
{
Console.Write(b[i]);
}
Console.ReadLine();
3).
const int r = 4;
const int c = 3;
int[,] t =
{
{20, 21, 22}, {30, 31, 32}, {40, 41, 42}, {50, 51, 52}
};
for (int i = 0; i < r; i++)
{
for (int j = 0; j < c; j++)
{
Console.WriteLine(“t[{0},{1}] = {2}”,
i, j, t[i, j]);
}
}
Console.ReadLine();
4).
String[] s = {“Learn”, “arrays”, “with”, “TalkIT.”};
Array.Sort(s);
foreach (object obj in s)
{
Console.WriteLine(“Value: {0}”, obj.ToString());
}
Array.Reverse(s);
foreach (object obj in s)
{
Console.WriteLine(“Value: {0}”, obj.ToString());
}
Console.ReadLine();
5).
const int rows = 2;
const int rowZero = 5;
const int rowOne = 6;
int[][] ja = new int[rows][];
ja[0] = new int[rowZero];
ja[1] = new int[rowOne];
ja[0][3] = 15;
ja[1][1] = 12;
ja[1][5] = 9;
for (int i = 0; i < rowZero; i++)
{
Console.WriteLine(ja[0][i]);
}
for (int i = 0; i < rowOne; i++)
{
Console.WriteLine(ja[1][i]);
}
Console.ReadLine();
}
}
Write your answers in the comments below and Share it. If you struggle just ask questions bellow and we will help you.