React is a popular client-side development library from Facebook. React makes it easy to create reusable components and render them in a web page or in a native mobile application.
This course focuses on React web development. We take a detailed look at how to create components using pure React and using JSX, and then take a detailed look at how to use Redux and Saga to manage state and asynchrony in large-scale applications.
Duration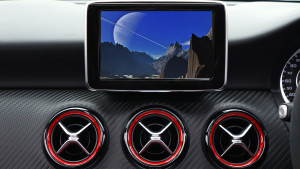
3 days
Prerequisites
Familiarity with HTML and JavaScript programming
What you’ll learn
- React architectural concepts
- Creating components via ES6 inheritance
- Creating components via stateless functional components
- Using JSX effectively
- React Router
- Redux and React
- Redux Saga
- Modular development and bundling using Webpack
Course details
Introduction to React
- What is React
- Essential ES6 language features for React
- Using the Babel transpiler
Getting Started With React
- Creating a simple React application
- Creating multiple React elements
- A data-driven approach
- Creating elements via ReactDOMFactories
Components
- Overview of React components
- Creating components via ES6 inheritance
- Creating functional stateless components
- Creating components via factories
JSX
- Overview of JSX
- A closer look at JSX Syntax
- Complete example of JSX
Creating Modular React Applications
- The need for modularity
- Example application using Webpack
Properties and State
- Specifying types for properties
- Working with ES6 classes
- Working with stateless functional components
- State management
Component Techniques
- Component lifecycle methods
- Practical example of lifecycle methods
- Optimizing UI updates
- Accessing child content in a component
React Router
- Overview of Single Page Applications
- React and SPAs
- Defining a router table
- Creating links
- Route parameters
Redux Store
- What is Redux
- Creating a Redux store
- Creating and dispatching actions
- Defining reducers
- Subscribing and unsubscribing to state changes
- Implementing action creators
- Composing functions
Redux and React
- Application structure
- Identifying actions and reducers
- Accessing state via context
- Defining container classes
- Simplifying containers via React Redux
Redux Saga
- Overview of Redux Saga
- ES6 generators
- Doing asynchronous work via Redux Saga
- Saga effects (take, put, call, fork, cancel, etc.)