Functional languages have some restrictions that will shock traditional coders. This follows from our previous blog on Why Bother with OOP?
Welcome to the latest news from TalkIT. These are my thoughts based on programming and training over the last 15 years. Please add your comments below.
Contents
So What Is It?
Some Key Principles
Why Bother?
Functional Coding in Action
Other Bits
So What Is It?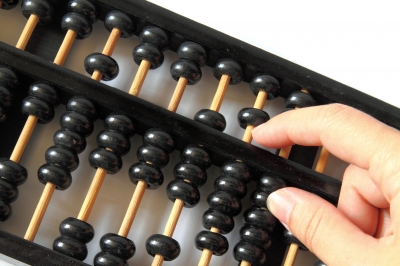
Functional Programming is a programming paradigm or toolset. As such it is programming language independent. It is radically different from other paradigms like Object Oriented (OO) and Imperative programming. It is firmly rooted in mathematics. Haskell is a popular contemporary functional language. C# 3.0, JavaScript and Java 8 are languages that allow functional, OO and imperative coding.
Functional coding has been used in industrial and commercial applications by a wide variety of organizations including FaceBook.
Here is a Wikipedia article on functional programming.
https://en.wikipedia.org/wiki/Functional_programming
Some Key Principles
A key principle is all computation is the execution of a function. What is a function? A function maps input parameters to outputs. Here are examples that take one and two inputs.
f(x) = x + 2
g(x,y) = 2x + y + 2
Let us call these functions.
f(3) = 3 + 2 = 5
g(4,5) = 2 * 4 + 5 + 2 = 15
The function cannot change the inputs. It is immutable or unchangeable. It encapsulates its data. This means there are no unpredictable side effects from a function call.
A function is a bit like a neuron in a human brain. These neurons work independently. There are fibres that take in signals from other neurons and fibres that take signals out. By wiring together billions of neurons, highly complex tasks are accomplished.
Functional languages have some restrictions that will shock traditional coders. There are no global variables or variable reassignments. Also loops with counter variables are banned.
Functional languages mostly use recursive functions to get things done. These functions repeatedly call themselves to implement repeated statements.
Why Bother?
Functional code provides a range of benefits.
- Ease of application maintenance – easy to find and fix bugs as each function is self contained.
- Code security – it is hard for a code hack to spread through functional code.
- Ease of parallel processing – it is safe to execute functions simultaneously in separate processors.
- Memory management – functional languages remove redundant data from memory.
Functional Coding in Action
Consider the problem of adding a series of integers. In Java we can use a familiar loop construct.
int total = 0;
for (int i = 0, i<=10, i++)
{
total = total + i;
}
In Haskell we cannot use variable reassignments or loops. But we can use a series of recursive function calls. The function sum[] calls itself to add up the integers. Here ns mean multiple n’s.
type
sum::[[int]] - - > int
sum[[n]] = n
sum[n:ns] = n + sum ns
This is a call to our function with four inputs.
sum [1,2,3,4]
It is worth mentioning that, as the problem is quite simple, both these snippets compile to similar native code. With similar performance.
Functional programming is a programming paradigm in C# that is frequently combined with object oriented programming. In C#, you will define something like:
books.Where(price<20).OrderBy(title).Take(10);
This is C# LINQ query – but it is also functional programming. LINQ is just one .Net class library that enables you to use functional programming concepts.
To find out more take a look at these tutorials in the TalkIT C# course.
- LINQ to Objects
- Delegates and Events
Other Bits
TalkIT has been very active on social media recently. We have been posting on coding and IT humour. Why don’t you connect with us on Twitter or FaceBook? You can follow all the latest news and let us know what you think.
Coding courses for people in the tech industry who want career opportunities & certifications. https://youtu.be/rQUTIyvoTqI
Creating a graphics program in C++. Learn coding in easy stages. Created by experts
Creating a Simple Python Game. Learn to code in easy steps. Created by experts. https://youtu.be/ptb1kjbjaA4
Time for a Career Opportunity? Coding courses with technical depth in industry languages. http://www.talk-it.biz .
Photos www.freedigitalphotos.net/
Thanks to Computerphile for the YouTube video
David Ringsell TalkIT 2017 ©